The Making of Foxhole Logistics Calculator
A technical breakdown of how and why the Foxhole Logistics Calculator came to be, and where it will end up. Follow the journey from design, to prototype, to a minimum viable product in production. You will learn the drawbacks of a logistics player in the game Foxhole, and why this utility was created for the community.
The Backstory
In the game Foxhole, I found myself in the muddy trenches defending against the Wardens, alongside other Colonial players. As the Wardens were pushing into the town of Longstone in the Westgate region, we found ourselves low of supplies, ammunition, and our morals low.
A lot of talk in chat had been made about requesting logistics to the region to help defend against the Wardens. And it made myself and several other players around me thing, what would it take to make our supplies.
Soon after I found myself in the pits, mining scrap to be processed into basic materials, a core item in crafting. During this time, I quickly came to realize that there wasn't too many quality-of-life tools for players who solely played as logistics.
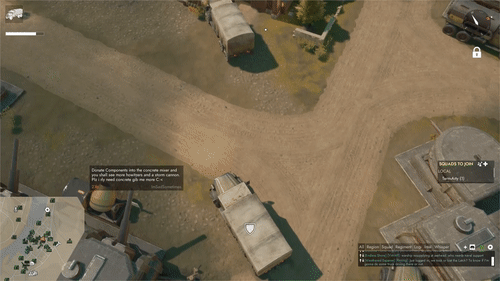
An issue that I had with that was when traveling long distances with these materials to create items, you would spend a long time looking at the game wiki calculating these costs before traveling to not waste time by being off on crafting prices.
Understanding the Problem
For a player to do logistics and deliver viable items to the front, they would have to go through several steps. This can be broken down into the process of gathering scrap, refining it, and then crafting the materials into items at factories that they got from the refinery. After waiting some time at the factory, they could collect these items and deliver them to the front.
The issue in my opinion lies between getting the basic materials together, and placing the factory order. This is because the map changes from war to war, and often times you will find the storage or refinery separated by a sizable distance to the factory. This time of travel can take between 5 minutes to 20 minutes depending on the time and place.
In order to be efficient with a time constrained role, you want to be precise and not wasteful. You definitely don't want to backtrack your route either to obtain more materials that you could be lacking.
The MPF Formula
A large portion of the website hinges on a mathematical formula the developers use for a discount found in the mass production factory (MPF). The only thing that gives any hint to how this formula works is a small description found within the items description cards when you're viewing them inside the MPF, which was -
10% discount per crate up to 50%
To help me figure this out and visualize the formula, I created a table that showed what values were at each step of adding a crate of items to the MPF order. The important fields to pay attention to are the Discount Cost Cumulative and Applied Discount. Applied discount is based on the individual base cost, in this case, 60 basic materials.
Number of Crates | Bmat Cost Cumulative | Discount Cost Cumulative | Applied Discount (60) | Discount |
---|---|---|---|---|
1 | 60 | 54 | 54 | 10% |
2 | 120 | 102 | 48 | 20% |
3 | 180 | 144 | 42 | 30% |
4 | 240 | 180 | 36 | 40% |
5 | 300 | 210 | 30 | 50% |
6 | 360 | 240 | 30 | 50% |
7 | 420 | 270 | 30 | 50% |
8 | 480 | 300 | 30 | 50% |
9 | 540 | 330 | 30 | 50% |
So We've Cracked the Formula?
Not exactly, take a close look at the table for explosive material costs for 120mm artillery shells.
Number of Crates | Emat Cost Cumulative | Discount Cost Cumulative | Applied Discount (15) | Discount |
---|---|---|---|---|
1 | 15 | 13.5 | 13.5 | 10% |
2 | 30 | 25.5 | 12 | 20% |
3 | 45 | 36 | 10.5 | 30% |
4 | 60 | 45 | 9 | 40% |
5 | 75 | 52.5 | 7.5 | 50% |
6 | 90 | 60 | 7.5 | 50% |
7 | 105 | 67.5 | 7.5 | 50% |
8 | 120 | 75 | 7.5 | 50% |
9 | 135 | 82.5 | 7.5 | 50% |
The results state that it should be 82.5 explosive materials. The two issues with that a full factory order in-game actually costs 79 explosive materials, and there is no such thing as 1/2 a material. This was clear evidence of a rounding point error, and I used Math.Floor()
on the cumulative cost per iteration to fix that. This can be seen on the function table of the cumulative costs for explosive materials.
Number of Crates | Formula | Applied Discount (15) |
---|---|---|
1 | 15 - (15 * .1) | 13 |
2 | 15 - (15 * .2) | 12 |
3 | 15 - (15 * .3) | 10 |
4 | 15 - (15 * .4) | 9 |
5 | 15 - (15 * .5) | 7 |
6 | 15 - (15 * .5) | 7 |
7 | 15 - (15 * .5) | 7 |
8 | 15 - (15 * .5) | 7 |
9 | 15 - (15 * .5) | 7 |
Total: 79 |
This function tabled helped me visualize that I needed to iterate through all crates keeping in minds the 10%-50% discount per crate while minding the Math.floor()
math function. This would take the number_of_crates
and resource_base_cost
as arguments and would return a resource_total_cost
. The loop variable would have to be checked if it is past 5 total crates to apply the flat discount rate of 50%. This is the math function that I would be using inside Foxhole Logi.
getItemDiscountCosts(number_of_crates, resource_base_cost) {
let resource_total_cost = 0
for(let i = 1; i <= number_of_crates; i++) {
if(i > 5) resource_total_cost += Math.floor(resource_base_cost - (resource_base_cost * (5/10)))
if(i <= 5) resource_total_cost += Math.floor(resource_base_cost - (resource_base_cost * (i/10)))
}
return resource_total_cost
}
Application Flow & Design Considerations
Before starting this project, I had to consider what language or framework I would choose. The options were standard html/css/js or a framework to help this development. I knew going into this project I would have to have a state management for global settings such as a factory or mass production, colonial or warden, and even a system for order management. I went with VueJS for its event emitters and the ability to add a store for state management.
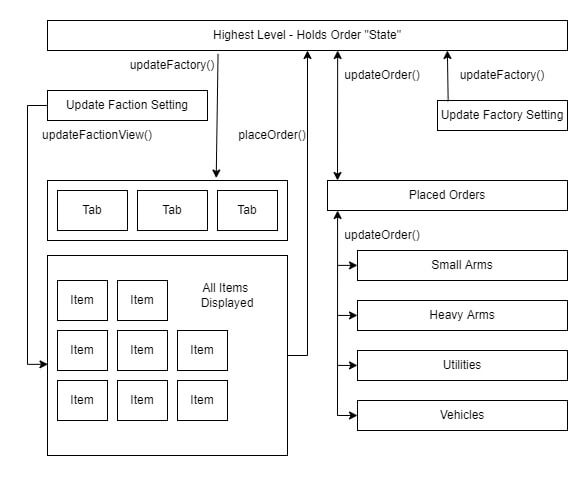
I ended up creating enough of a minimum viable product with only event emitters, but would later find out that this is hard to maintain and expand on functionality as it made a mess of my code.
There's a fake "state" found at the root of the page, and is sent information via emitters on items, settings, removing an order, and adding an order. These events are processed at the root component, and are passed down to components via props.
If I were to recreate an application to this size again, I would proceed with using Vuex as a state management instead of relying on emitters/props. Although messy, it was a learning experience as I had never built with framework with the mindset of reactive programming.
Game Data
Unlike the War Api provided by Clapfoot, which gives information about in-game activities to create applications like FoxholeStats. There are no APIs to obtain information about item data such as names, descriptions, costs, and more.
This is why I meticulously collected, recorded, and maintain a single JSON file containing this information for the general public which you can view on the website.
Menu Structure & Design
Since I did not plan to initially include localization for other languages due to costs, the next thing that was needed was a system to replicate in-game menu structure with their icons. This way the user will be able to recognize these icons and be able to more reliably use this application without too much need for relying on text.
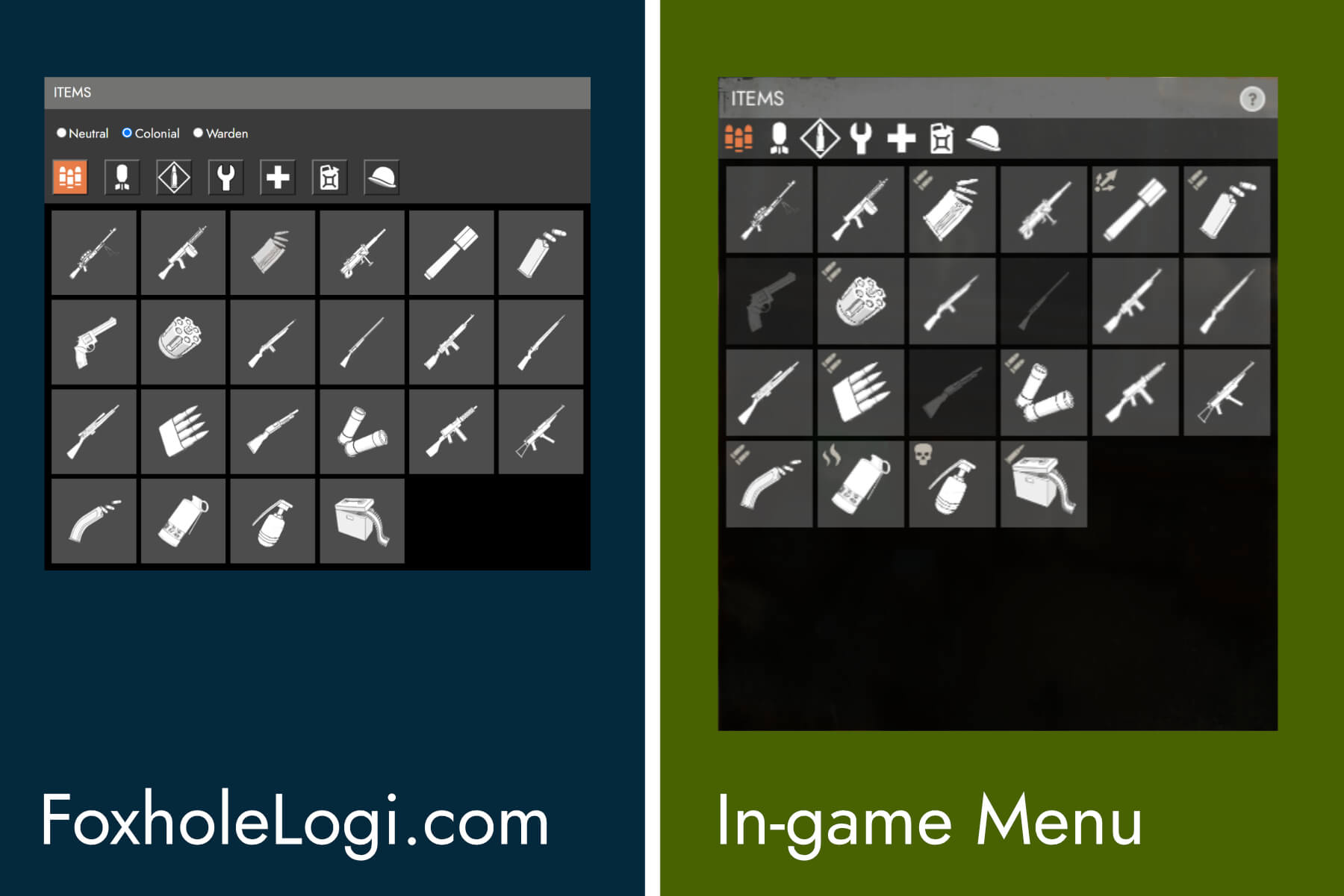
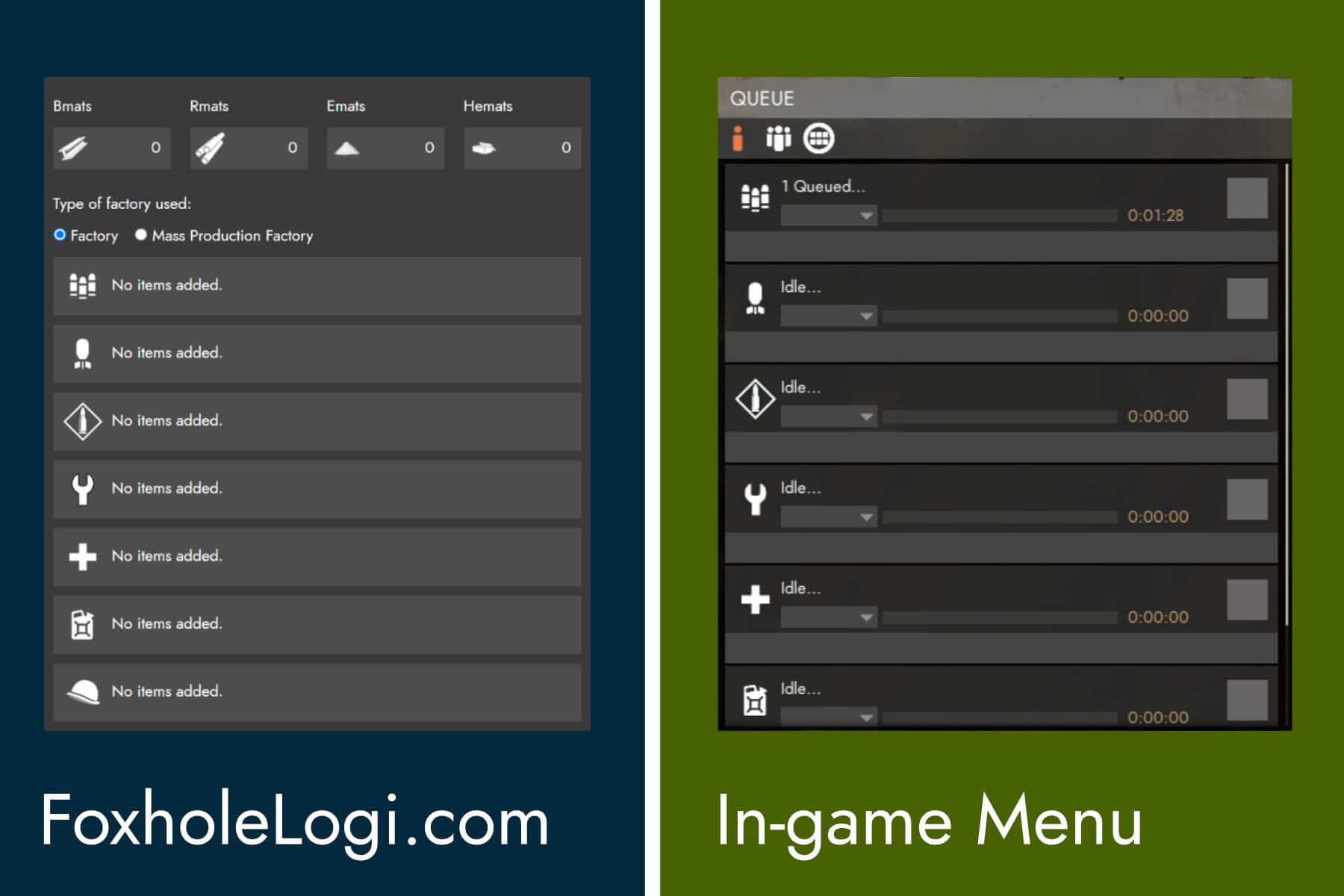
Take Away from Project
What I learned
Overall, I'm happy with myself and am proud of my work. It was a learning experience as it was my first time working with VueJS or any reactive programming language. Although some mistakes were made, and the minimal viable product's code is a bit messy to maintain, I'm still very proud of FoxholeLogi.
How I can Improve
Here's some things that I will be working on to further improve this application.
- Focus on mobile development
- Optimizations - component loading / file loading
- Localization
- Item Search Bar
- Push Notification for order time estimation
- Breakdown price to crates
- A lot more not listed here